"Lab Static Method"
package s9226307;
public class s9226307
{
private static int prenum = 1;
private static int num = 1;
private static int sum = 1;
public static int next()
{
sum = sum + prenum;
prenum = num;
num = sum;
return sum;
}
}
Lab Sorting
package s9226307;
public class Sorting
{
public static void main(String args[])
{
double[] number= {4, 3, 6, 7, 5,4.3,8,9.9,11,2};
int x,y;
double z;
for(x=0;x<10;x++) y="0;y<10;y++)">number[y])
{
z=number[x];
number[x]=number[y];
number[y]=z;
}
}
}
for(x=0;x<10;x++)
{
System.out.print(" "+number[x]+" ");
}
System.out.println("");
System.out.println("由大到小");
System.exit(0);
}
}
"Lab Recursive Method"
package s9226307;
public class s9226307 {
public static void main(String[] args) {
System.out.println(""+recursive(3,1,2,3));
}
public static int recursive(int n,int src,int aux,int des)
{
if(n==1)
{
System.out.println("Move " +1+ " from " +src+ " to "+des);
}
else
{
recursive(n-1, src, des, aux);
System.out.println("Move " +n+ " from " +src+ " to "+des);
recursive(n - 1, aux, src, des);
}
return 0;
}
}
"Lab Hanoi Tower"
package s9226307;
public class s9226307 {
public static void main(String[] args) {
System.out.println(""+recursive(3,1,2,3));
}
public static int recursive(int n,int src,int aux,int des)
{
if(n==1)
{
System.out.println("Move " +1+ " from " +src+ " to "+des);
}
else
{
recursive(n-1, src, des, aux);
System.out.println("Move " +n+ " from " +src+ " to "+des);
recursive(n - 1, aux, src, des);
}
return 0;
}
}
"Lab Recursive Method"
package s9226307;
import javax.swing.JOptionPane;
public class Recursion
{
public static void main(String[] arg)
{
String PString=JOptionPane.showInputDialog("Enter a number n=");
int i=Integer.parseInt(PString);
System.out.println( Recursion.comput(i));
System.exit(0);
}
public static void sum(int i)
{
if(i == 1)
System.out.print(1);
else{
sum(i - 1);
System.out.print(" + "+ i * i);
}
}
public static int comput(int i)
{
if(i == 1)
return 1;
else
return (comput(i - 1) + i * i);
}
}
"Lab Sorting"
public class ArrayOfLength
{
public static void main (String[] arges)
{
int [] lenght= {12,54,66,88,99};
for(int i=0;i<5;i++)
{
for(int j=0;j<5;j++)
{
if(lenght[i]>lenght[j])
{
int a;
a=lenght[i];
lenght[i]=lenght[j];
lenght[j]=a;
}
}
}
for(int i=0;i<5;i++)
{
System.out.println(lenght[i]);
}
}
}
Homework 5/29
package s9226307;
public class Square
{
public static void main(String[] args)
{
int i , j ;
int box;
int[]age={9,7,10,1,3};
for(i=0;i<5;i++) j="i+1;j<5;j++)">age[i])
{
box=age[i];
age[i]=age[j];
age[j]= box ;
}
}
System.out.println(age[i]*age[i]);
}
}
}
====================
100
81
49
9
1
Lab 13
package s9226307;
public class s92263407 {
public static void main(String[] args){
int[] number={1,6,33,44,79,6,99,125,389};
int x,y,z;
for(x=0;x less than number.length;x++)
{
for(y=x+1;y less than number.length;y++)
{
if (number[x] greater than number[y])
{
z=number[x];
number[x]=number[y];
number[y]=z;
}
}
}
for(x=0;x less than number.length;x++)
{
System.out.println(""+number[x]);
}
}
}
Homework 5/8
package Temperature;public class Temperature {private double value;private char scale;public Temperature() {this.value = 0;this.scale = 'C';}public Temperature(double value) {this.value = value;this.scale = 'C';}public Temperature(char scale) {this.value = 0;if (scale == 'f' scale == 'F') {this.scale = 'F';} else if (scale == 'c' scale == 'C') {this.scale = 'C';} else {System.out.println("Error Scale!! scale set C");this.scale = 'C';}}public Temperature(double value, char scale) {this.value = value;if (scale == 'f' scale == 'F') {this.scale = 'F';} else if (scale == 'c' scale == 'C') {this.scale = 'C';} else {System.out.println("Error Scale!! scale set C");this.scale = 'C';}}public double getTempC() {double rtnTemp = 0;if (scale == 'C') {rtnTemp = value;} else if (scale == 'F') {rtnTemp = (value - 32) * 5 / 9;}return rtnTemp;}public double getTempF() {double rtnTemp = 0;if (scale == 'F') {rtnTemp = value;} else if (scale == 'C') {rtnTemp = (value * 9 / 5) + 32;}return rtnTemp;}public void setValue(double value) {this.value = value;}public void setScale(char scale) {if (scale == 'f' scale == 'F') {this.scale = 'F';} else if (scale == 'c' scale == 'C') {this.scale = 'C';} else {System.out.println("Error Scale!!scale no change");}}public void setTemp(double value, char scale) {if (scale == 'f' scale == 'F') {this.scale = 'F';} else if (scale == 'c' scale == 'C') {this.scale = 'C';} else {System.out.println("Error Scale!!scale no change");}this.value = value;}public boolean isEquals(Temperature otherTemp) {if (this.scale == 'C' && this.value == otherTemp.getTempC()) {return true;} else if (this.scale == 'F' && this.value == otherTemp.getTempF()) {return true;} else {return false;}}public boolean isGreaterThan(Temperature otherTemp) {if (this.scale == 'C' && this.value >= otherTemp.getTempC()) {return true;} else if (this.scale == 'F' && this.value >= otherTemp.getTempF()) {return true;} else {return false;}}public boolean isLessThan(Temperature otherTemp) {if (this.scale == 'C' && this.value <= otherTemp.getTempC()) {return true;} else if (this.scale == 'F' && this.value <= otherTemp.getTempF()) {return true;} else {return false;}}public String toString() {return Double.toString(value) + scale;}}class TemperatureDemo {public static void main(String[] args) {Temperature iceC = new Temperature(0.0, 'C');Temperature iceF = new Temperature(32.0, 'F');Temperature fireC = new Temperature(100.0);fireC.setScale('C');Temperature fireF = new Temperature(212.0);fireF.setScale('F');Temperature coldC = new Temperature();coldC.setTemp( -40.0, 'C');Temperature coldF = new Temperature();coldF.setScale('F');coldF.setValue( -40.0);System.out.println("when TemperatureC = " + iceC );System.out.println("then TemperatureF = " + iceF );System.out.println("when TemperatureC = " + fireC );System.out.println("then TemperatureF = " + fireF );System.out.println("when TemperatureC = " + coldC );System.out.println("then TemperatureF = " + coldF );}}
Lab Static Method, Part II
package s9226307;import java.io.*;public class s9226307 {public static void main(String agrs[]) throws IOException{double num1,num2,num3,num4,sum,sum1;String str,str2,str3,str4;BufferedReader buf,buf2,buf3,buf4;System.out.println("請輸入4個數字,前面兩個為實數後面兩個為複數:");buf=new BufferedReader(new InputStreamReader(System.in));str=buf.readLine();num1=Double.parseDouble(str);buf2=new BufferedReader(new InputStreamReader(System.in));str2=buf2.readLine();num2=Double.parseDouble(str2);buf3=new BufferedReader(new InputStreamReader(System.in));str3=buf3.readLine();num3=Double.parseDouble(str3);buf4=new BufferedReader(new InputStreamReader(System.in));str4=buf4.readLine();num4=Double.parseDouble(str4);sum=num1+num2;sum1=num3+num4;System.out.println
("你輸入的數字為:"+num1+"+"+num3+"i ,"+num2+"+"+num4+"i");System.out.println
("總合為:"+sum+"+"+sum1+"i");
}
}
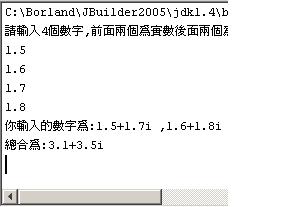
Cookie
Lab Counter II
package s9226307;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class s9226307 {
private int number;
public s9226307()
{
}
public void readInput() throws IOException
{
BufferedReader keyboard = new BufferedReader(new InputStreamReader(System. in));
System.out.println("Enter a number.");
System.out.println("Do not use a comma.");
number = keyboard.read();
}
public void reset()
{
number = 0 ;
}
public void inc()
{
number = number + 1 ;
}
public void dec()
{
number = number - 1 ;
}
public void writeOutput()
{
System.out.println("counter number is " + number);
}
public boolean equals(s9226307 otherCounter)
{
return this.number == otherCounter.number;
}
public void resetToZero()
{
number = 0;
}
public String toString()
{
return Integer.toString(number) ;
}
}
-------------------------------------------------------------------------------------
package s9226307;
import java.io.IOException;
public class ccc
{
public static void main(String[] args) throws IOException
{
s9226340 number1 = new s9226307(),
number2 = new s9226307();
number1.inc();
number2.inc();
if (number1.equals(number2))
{
System.out.println( number1 + " is equal to " + number2);
}
else
{
System.out.println( number1 + " is not equal to " + number2);
}
number2.inc();
number1.dec();
if (number1.equals(number2))
{
System.out.println( number1 + " is equal to " + number2);
}
else
{
System.out.println( number1 + " is not equal to " + number2);
}
}
}
Lab Java Constructor
package s9226307;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class Date
{
private String month;
private int day;
private int year;
public Date()
{
month = "January";
day = 1;
year = 1000;
}
public Date (int monthInt, int day, int year)
{
setDate(monthInt,day,year);
}
public Date (String monthString, int day, int year)
{
setDate(monthString,day,year);
}
public Date(int year)
{
setDate (1,1,year);
}
public Date(Date aDate)
{
if (aDate==null)
{
System.out.println("Fatal Error");
System.exit(0);
}
month=aDate.month;
day=aDate.day;
year=aDate.year;
}
public void setDate(int monthInt, int day, int year)
{
if (dateOK(monthInt, day, year))
{
this.month = monthString(monthInt);
this.day = day;
this.year = year;
}
else
{
System.out.println("Fatal Error");
System.exit(0);
}
}
public void setDate(String monthString, int day, int year)
{
if (dateOK(monthString, day, year))
{
this.month = monthString;
this.day = day;
this.year = year;
}
else
{
System.out.println("Fatal Error");
System.exit(0);
}
}
public void setDate(int year)
{
setDate(1, 1, year);
}
private boolean dateOK(int monthInt, int dayInt, int yearInt)
{
return ( (monthInt >= 1) &&(monthInt <= 12) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999));
}
private boolean dateOK(String monthString, int dayInt, int yearInt)
{
return (monthOK(monthString) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999));
}
private boolean monthOK(String month)
{
return (month.equals("January") || month.equals("February") || month.equals("March") ||
month.equals("April") || month.equals("May") || month.equals("June") ||
month.equals("July") || month.equals("August") || month.equals("September") ||
month.equals("October") ||month.equals("November") || month.equals("December"));
}
public void readInput() throws IOException
{
boolean tryAgain = true;
BufferedReader keyboard = new BufferedReader(new InputStreamReader(System. in));
while (tryAgain)
{
System.out.println("Enter month, day, and year.");
System.out.println("Do not use a comma.");
String monthInput = keyboard.readLine();
int dayInput = keyboard.read();
int yearInput = keyboard.read();
if (dateOK(monthInput, dayInput, yearInput))
{
setDate(monthInput, dayInput, yearInput);
tryAgain = false;
}
else
System.out.println("Illegal date. Reenter input.");
}
}
public void writeOutput()
{
System.out.println(month + " " + day + ", " + year);
}
public void setMonth(int month)
{
if ( (month <= 0) || (month > 12))
{
System.out.println("Fatal Error");
System.exit(0);
}
else
this.month = monthString(month);
}
public void setMonth(String month)
{
this.month = month;
}
public void setDay(int day)
{
if ( (day <= 0) || (day > 31))
{
System.out.println("Fatal Error");
System.exit(0);
}
else
this.day = day;
}
public void setYear(int year)
{
if ( (year <= 1000) || (year >= 9999))
{
System.out.println("Fatal Error");
System.exit(0);
}
else
this.year = year;
}
public boolean equals(Date otherDate)
{
return ( (month.equalsIgnoreCase(otherDate.month))
&& (day == otherDate.day) && (year == otherDate.year));
}
public boolean precedes(Date otherDate)
{
return ( (year < otherDate.year) ||
(year == otherDate.year && getMonth() < otherDate.getMonth()) ||
(year == otherDate.year && month.equals(otherDate.month) &&
day < otherDate.day));
}
public String toString()
{
return (month + " " + day + ", " + year);
}
public int getDay()
{
return day;
}
public int getYear()
{
return year;
}
public int getMonth()
{
if (month.equalsIgnoreCase("January"))
return 1;
else if (month.equalsIgnoreCase("February"))
return 2;
else if (month.equalsIgnoreCase("March"))
return 3;
else if (month.equalsIgnoreCase("April"))
return 4;
else if (month.equalsIgnoreCase("May"))
return 5;
else if (month.equals("June"))
return 6;
else if (month.equalsIgnoreCase("July"))
return 7;
else if (month.equalsIgnoreCase("August"))
return 8;
else if (month.equalsIgnoreCase("September"))
return 9;
else if (month.equalsIgnoreCase("October"))
return 10;
else if (month.equalsIgnoreCase("November"))
return 11;
else if (month.equalsIgnoreCase("December"))
return 12;
else
{
System.out.println("Fatal Error");
System.exit(0);
return 0;
}
}
private String monthString(int monthNumber)
{
switch (monthNumber)
{
case 1:
return "January";
case 2:
return "February";
case 3:
return "March";
case 4:
return "April";
case 5:
return "May";
case 6:
return "June";
case 7:
return "July";
case 8:
return "August";
case 9:
return "September";
case 10:
return "October";
case 11:
return "November";
case 12:
return "December";
default:
System.out.println("Fatal Error");
System.exit(0);
return "Error";
}
}
}
=================================
package s9226307;
public class s9226307 {
public s9226307 () {
}
public static void main(String[] args)
{
Date date1 = new Date("December", 16, 1770),
date2 = new Date(1, 27, 1756),
date3 = new Date(1882),
date4 = new Date();
Date birthday = new Date("January",1,2000);
birthday.setDate("February",1,2000);
birthday = new Date("March",1,2000);
System.out.println("Whose birthday is "+ date1+ "?");
System.out.println("Whose birthday is "+ date2+ "?");
System.out.println("Whose birthday is "+ date3+ "?");
System.out.println("The default date is "+ date4+ ".");
}
}
Homework 05-01-2006 Lab Counter
package cccc;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class Counter
{
private int count;
public void readInput() throws IOException
{
boolean tryAgain = true;
BufferedReader keyboard = new BufferedReader(new InputStreamReader(System. in));
System.out.println("Enter a number.");
while (tryAgain)
{
String count1 = keyboard.readLine();
count = Integer.parseInt(count1);
if (count >= 0)
tryAgain = false;
else
System.out.println("Illegal date. Return input.");
}
}
public void reset()
{
count = 0;
}
public void inc()
{
count = count + 1;
}
public void dec()
{
count = count - 1;
}
public void output()
{
System.out.println(count);
}
}
//=================================
package cccc;
import java.io.IOException;
public class CounterDemo
{
public static void main(String[] args) throws IOException
{
Counter counter= new Counter();
counter.readInput();
counter.inc();
counter.output();
counter.inc();
counter.output();
counter.dec();
counter.output();
counter.reset();
counter.output();
}
}
Lab Java Constructor
package s9226307;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class Date
{
private String month;
private int day;
private int year;
public Date(){
month="January";
day=1;
year=1000;
}
public Date(int monthInt, int day, int year){
setDate(monthInt,day,year);
}
public Date(String monthString, int day, int year){
setDate(monthString,day,year);
}
public Date(int year){
setDate(1,1,year);
}
public Date(Date aDate){
if (aDate==null){
System.out.println("Fatal Error.");
System.exit(0);
}
month=aDate.month;
day=aDate.day;
year=aDate.year;
}
public void setDate(int monthInt, int day, int year)
{
if (dateOK(monthInt, day, year))
{
this.month = monthString(monthInt);
this.day = day;
this.year = year;
}
else
{
System.out.println("Fatal Error");
System.exit(0);
}
}
public void setDate(String monthString, int day, int year)
{
if (dateOK(monthString, day, year))
{
this.month = monthString;
this.day = day;
this.year = year;
}
else
{
System.out.println("Fatal Error");
System.exit(0);
}
}
public void setDate(int year)
{
setDate(1, 1, year);
}
private boolean dateOK(int monthInt, int dayInt, int yearInt)
{
return ( (monthInt >= 1) &&(monthInt <= 12) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999));
}
private boolean dateOK(String monthString, int dayInt, int yearInt)
{
return (monthOK(monthString) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999));
}
private boolean monthOK(String month)
{
return (month.equals("January") || month.equals("February") || month.equals("March") ||
month.equals("April") || month.equals("May") || month.equals("June") ||
month.equals("July") || month.equals("August") || month.equals("September") ||
month.equals("October") ||month.equals("November") || month.equals("December"));
}
public void readInput() throws IOException
{
boolean tryAgain = true;
BufferedReader keyboard = new BufferedReader(new InputStreamReader(System. in));
while (tryAgain)
{
System.out.println("Enter month, day, and year.");
System.out.println("Do not use a comma.");
String monthInput = keyboard.readLine();
int dayInput = keyboard.read();
int yearInput = keyboard.read();
if (dateOK(monthInput, dayInput, yearInput))
{
setDate(monthInput, dayInput, yearInput);
tryAgain = false;
}
else
System.out.println("Illegal date. Reenter input.");
}
}
public void writeOutput()
{
System.out.println(month + " " + day + ", " + year);
}
public void setMonth(int month)
{
if ( (month <= 0) || (month > 12))
{
System.out.println("Fatal Error");
System.exit(0);
}
else
this.month = monthString(month);
}
public void setMonth(String month)
{
this.month = month;
}
public void setDay(int day)
{
if ( (day <= 0) || (day > 31))
{
System.out.println("Fatal Error");
System.exit(0);
}
else
this.day = day;
}
public void setYear(int year)
{
if ( (year <= 1000) || (year >= 9999))
{
System.out.println("Fatal Error");
System.exit(0);
}
else
this.year = year;
}
public boolean equals(Date otherDate)
{
return ( (month.equals(otherDate.month))
&& (day == otherDate.day) && (year == otherDate.year));
}
public boolean precedes(Date otherDate)
{
return ( (year < otherDate.year) ||
(year == otherDate.year && getMonth() < otherDate.getMonth()) ||
(year == otherDate.year && month.equals(otherDate.month) &&
day < otherDate.day));
}
public String toString()
{
return (month + " " + day + ", " + year);
}
public int getDay()
{
return day;
}
public int getYear()
{
return year;
}
public int getMonth()
{
if (month.equalsIgnoreCase("January"))
return 1;
else if (month.equalsIgnoreCase("February"))
return 2;
else if (month.equalsIgnoreCase("March"))
return 3;
else if (month.equalsIgnoreCase("April"))
return 4;
else if (month.equalsIgnoreCase("May"))
return 5;
else if (month.equals("June"))
return 6;
else if (month.equalsIgnoreCase("July"))
return 7;
else if (month.equalsIgnoreCase("August"))
return 8;
else if (month.equalsIgnoreCase("September"))
return 9;
else if (month.equalsIgnoreCase("October"))
return 10;
else if (month.equalsIgnoreCase("November"))
return 11;
else if (month.equalsIgnoreCase("December"))
return 12;
else
{
System.out.println("Fatal Error");
System.exit(0);
return 0;
}
}
private String monthString(int monthNumber)
{
switch (monthNumber)
{
case 1:
return "January";
case 2:
return "February";
case 3:
return "March";
case 4:
return "April";
case 5:
return "May";
case 6:
return "June";
case 7:
return "July";
case 8:
return "August";
case 9:
return "September";
case 10:
return "October";
case 11:
return "November";
case 12:
return "December";
default:
System.out.println("Fatal Error");
System.exit(0);
return "Error";
}
}
}
-------------------------------------------------------------------------------
package s9226307;
public class s9226307 {
public s9226340() {
}
public static void main(String[] args) {
Date date1 = new Date("December",16,1770),
date2 = new Date(1,27,1756),
date3 = new Date(1882),
date4 = new Date();
System.out.println("Whose birthday is "+ date1 +"?");
System.out.println("Whose birthday is "+ date2 +"?");
System.out.println("Whose birthday is "+ date3 +"?");
System.out.println("Whose default date is"+ date4 +".");
}
}