Lab Exponential Funtion
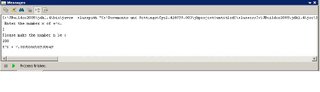
package s9226307;
import java.io.*;
public class s9226307
{
public static void main(String[] args) throws IOException
{
BufferedReader keyin= new BufferedReader(new InputStreamReader(System.in));
System.out.println(" Enter the number x of e^x.");
int x = Integer.parseInt(keyin.readLine());
System.out.println("Please make the number n be :");
int n = Integer.parseInt(keyin.readLine());
double p,q,r,s,a,sum=0;
for(p=0;p<=n;p++) { r=1; for(q=p;q>0;q--)
r=r*q;
a=1;
for(s=p;s>0;s--)
a=a*x;
sum=sum+(a/r);
}
System.out.println("e^x = "+sum);
}
}
Homework 3-20-2006
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class Fibonacci
{
public static void main(String[] args) throws IOException
{
int i;
double f0 = 0, f1 = 1, f2 = 0, ratio = 0;
for (i = 1; i <= 100; i++)
{
f2 = f0 + f1;
ratio = f2 / f1;
f0 = f1;
f1 = f2;
System.out.println(i+"\t"+f2+"\t"+" ratio is "+ratio);
}
}
}
Lab 5
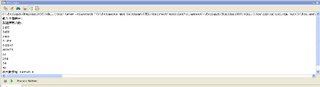
package s9226307;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class s9226307{
public s9226307(){
}
public static void main(String[] args)throws IOException
{
BufferedReader keyboard= new BufferedReader(new InputStreamReader(System.in));
System.out.println("輸入十個數字:");
System.out.println("我選擇最大的.");
double max=-1,min=-1,num=0;
int n;
String input="";
for(n=1;n<=10;n++) { input = keyboard.readLine(); num = Double.parseDouble(input); if (num>=max)
max = num;
if(num<=min)
min = num;
}
System.out.println("最大數字是 "+max);
}
}
Homework 3/13
Tax
package s9226307;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class s9226307 {
public s9226307() {
}
public static void main(String[] args)throws IOException
{
BufferedReader keyboard= new BufferedReader(new InputStreamReader(System.in));
double wealth,tax;
System.out.println("Enter your wealth:");
String podString = keyboard.readLine();
wealth=Integer.parseInt(podString);
if(wealth<=370000)
tax=0;
else if((wealth>370000)&&(wealth<=990000))
tax=(wealth-370000)*0.13+25900;
else if((wealth>)&&(wealth<=1980000))
tax=(wealth-990000)*0.21;+105100
else if((wealth>)&&(wealth<=3720000))
tax=(wealth-1980000)*0.3;+283300
else{
tax=(wealth-655300)*0.4;
}
System.out.println("Tax due=$"+tax);
}
}
find the max and min
package s9226307;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class s9226307{
public s9226307(){
}
public static void main(String[] args)throws IOException
{
BufferedReader keyboard= new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter some nonnegative numbers:");
System.out.println("Mark the end with a negaive number.");
System.out.println("I will compare Max and min");
double max=-1,min=-1,num=0;
int count=0;
String input="";
while(num >= 0)
{
input = keyboard.readLine();
num = Double.parseDouble(input);
if ((max==-1)||(num>=max)&&num>=0)
max = num;
if((min==-1)||(num<=min)&&num>=0)
min = num;
count++;
}
if (count==0)
System.out.println("No numbers entered.");
else
{
System.out.println("There are "+count+" numbers read.");
System.out.println("The max is "+max+" and the min is "+min);
}
}
}
Lab Scanner (lab 4)
程式碼:
package s9226307;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class s9226307 {
public s9226307() {
}
public static void main(String[] args) throws IOException
{
BufferedReader keyboard= new BufferedReader(new InputStreamReader(System.in));
String inputString = keyboard.readLine();
System.out.println("Enter number og pods");
String podString = keyboard.readLine();
int numberOfPods = Integer.parseInt(podString);
System.out.println("Enter number of peas in a pod:");
String peaString = keyboard.readLine();
int peasPerPod = Integer.parseInt(peaString);
int totalNumberOfPeas = numberOfPods*peasPerPod;
System.out.println(numberOfPods + " pods and ");
System.out.println(peasPerPod + " Peas per pod. ");
System.out.println("The total nimber of peas " + totalNumberOfPeas);
}
}
java JOptionpane
import javax.swing.JOptionPane;
public class Untitled1
{
public static void main(String[] args)
{
String myString=JOptionPane.showInputDialog("Enter a number: ");
int myNumber = Integer.parseInt(myString);
System.out.println("The number is "+ myNumber);
System.exit(0);
}
}
Project 5
程式:
package project5;
public class project5
{
public static void main(String[] args)
{
String sentence="I hate you.";
int position= sentence.indexOf("hate");
String ending=sentence.substring(position + "hate".length());
System.out.println("The line of text to be changed is");
System.out.println(sentence);
sentence=sentence.substring(0, position) + "love" + ending;
System.out.println("I have rephrased that line to read:");
System.out.println(sentence);
}
}
結果:
The line of text to be changed is
I hate you.
I have rephrased that line to read:
I love you
Lab Display1.7
程式:
package s9226307;
public class s9226307
{
public static void main(String[] args)
{
String sentence="I hate text processing!";
int position= sentence.indexOf("hate");
String ending=sentence.substring(position + "hate".length());
System.out.println("012344567890123456789012");
System.out.println(sentence);
System.out.println("The word \"hate\" starts at index " + position);
sentence=sentence.substring(0, position) + "adore" + ending;
System.out.println("The changed string is:");
System.out.println(sentence);
}
}
結果:
012344567890123456789012
I hate text processing!
The word "hate" starts at index 2
The changed string is:
I adore text processing!
HOMEWORK 2
package s9226307;
public class s9226307 {
public s9226307() {
}
public static void main(String[] args){
double calories;
int METS;
METS=10*30+8*30+6*60;
calories=METS*0.0175*150/2.2;
System.out.println("The total number of calories burned of a person who's weights is 150 pound "+calories);
}
}